1. General
-
Definition: constraints are imposed on general trees:
-
Each node can have at most two subtrees, that is, there are no binary trees Degree greater than 2 Node of
-
Subtree has Left right order Division of
-
There are 5 forms:
- Full binary tree and complete binary tree (delete nodes from right to left at the bottom of full binary tree)
2. Important characteristics
- Binary tree, in the second i Layers have at most 2i-1 Nodes
- Depth is k A binary tree has at most 2k-1 Nodes
- Height (or depth) is K A complete binary tree of at least 2k-2 individual leaf node
- Of nonempty binary tree Leaf node number Equal to 2 degrees The number of nodes plus 1, that is, n0 = n2 + 1
n1 of a complete binary tree can only be 0 or 1
- One degree is m If the node with degree 1 is n1, the node with degree 2 is n2,..., and the number of nodes with degree m is nm, then the number of leaf nodes is n0**= 1 + n2****+ 2n3 * * * * +... + (m-1)nm**
- Complete binary tree with n nodes, depth log2n + 1
- Number property: complete binary tree with n nodes (its depth is log2n + 1), number each node from top to bottom and from left to right (1~n), then: if i is the number of a node:
- If I is not equal to 1, the parent node number of a is: ⌊ i/2 ⌋
- If 2I ≤ n, then the left child number of a is 2i; If * * 2I > n * *, then a** No left child * *;
- If * * 2I + 1 ≤ n * *, the right child number of a is** 2i + 1**; If** 2I + 1 > n * *, then a** No right child * *;
1. Sequential storage
- use array To store data elements
- From the storage point of view, this sequential storage structure is only applicable to Complete binary tree
Because in the worst case, a depth of k And only k A single tree with two nodes (there is no node with degree 2 in the tree), but it needs a length of 2k-1 A one-dimensional array of.
2. Chain storage
- with Linked list Store data elements and the relationship between data elements.
1. Determine the binary tree from the traversal sequence
- from First order and middle order can be determined
- from Post order and middle order can be determined (but note that the last post order is the root and the next is the right subtree root)
- from Hierarchy and middle order can be determined
2. Estimate the binary tree according to the traversal sequence
- Preamble Ergodic sequence sum Post order Ergodic sequence identical Tree: only root node
- Preamble Ergodic sum Middle order ergodic identical Binary tree: all nodes have no left subtree (right single branch tree)
- Middle order Ergodic sum Post order ergodic identical Binary tree: all nodes have no right subtree (left single branch tree)
- Preorder ergodic sum Post order ergodic contrary Binary tree: no left subtree or no right subtree (only one leaf node) with a height equal to the number of nodes
- Preamble Ergodic sum Middle order ergodic contrary Binary tree: all nodes have no right subtree (left single branch tree)
- Middle order Ergodic sum Post order ergodic contrary Binary tree: all nodes have no left subtree (right single branch tree)
3. Traversal and tree building code
- Construction of binary tree
- Depth first traversal (first order, middle order and last order)
- Breadth first traversal (first order, second order)
/* BitTree.java */ package com.java.tree; import java.util.LinkedList; import java.util.Queue; /** * Created by Jaco.Young. * 2018-06-13 18:26 */ public class BitTree { //Represents the root node of a tree uniquely determined by preorder and inorder private TreeNode root; /** * Methods provided to external calls * The character array pre represents the preorder traversal sequence, and mid represents the medium order traversal sequence */ public void build(char[] pre, char[] mid){ //Assign the root node of the created tree to root root = buildTree(pre,0, pre.length-1, mid, 0, mid.length-1); } /** * Prerequisite: there are no duplicate elements in the tree * A method of constructing binary tree from preorder traversal sequence and middle order traversal sequence * In the process of building a tree, we always divide the sequence into left subtree and right subtree * lPre,rPre And lMid, rMid, respectively, indicate which part of the preorder and middle order to create a tree */ private TreeNode buildTree(char[] pre, int lPre, int rPre, char[] mid, int lMid, int rMid){ //In the preorder traversal sequence, find the root node of the current tree char root = pre[lPre]; //In the middle order traversal sequence, the position in the middle order is found according to the root node in the first order int rootIndex = getRootIndex(mid, lMid, rMid, root); //If not found, the given parameter is abnormal if(rootIndex == -1){ throw new IllegalArgumentException("Illegal Argument!"); } //Calculate the number of left and right subtrees of the current tree //Entire middle order sequence: [left subtree (lmid) root (rootindex) right subtree (rMid)] //Left subtree [lMid,rootIndex-1] int lNum = rootIndex - lMid; //rootIndex-1 -lMid + 1 //Right subtree [rootIndex+1,rMid] int rNum = rMid - rootIndex; //rMid - (rootIndex + 1) + 1 //Start to build the left and right subtrees of the current root node //First build the left subtree TreeNode lchild; //As the root node of the left subtree //The tree with the current node as the root has no left subtree if(lNum == 0){ lchild = null; }else{ //At present, the left subtree of this tree is still a tree. Recursively construct the left subtree of this tree //Let x be the subscript of the last element of the left subtree in the current tree precedence, then: x - (lpre + 1) = lNum //Get: x = lPre + lNum lchild = buildTree(pre, lPre + 1, lPre+lNum, mid, lMid, rootIndex - 1); } //Constructing right subtree TreeNode rchild; if(rNum == 0){ rchild = null; }else{ //The right subtree of the current node still contains many nodes, and its right subtree needs to be constructed recursively rchild = buildTree(pre, lPre + lNum + 1, rPre, mid, rootIndex + 1, rMid); } //Construct a complete binary tree return new TreeNode(root,lchild,rchild); } //In the middle order traversal sequence, the position in the middle order is found according to the root node in the first order private int getRootIndex(char[] mid, int lMid, int rMid, char root) { for(int i = lMid; i <= rMid; i++){ if(mid[i] == root){ return i; } } return -1; } //Structure of each node of binary tree private class TreeNode{ //Data stored in nodes char item; //Point to left child node > **Java Network disk: pan.baidu.com/s/1MtPP4d9Xy3qb7zrF4N8Qpg > Extraction code: 2 p8n** ### last After all, I've been working for so long. Besides tuhu's round, I also interviewed many big factories in July and August. For example, Ali, hungry, meituan and Didi, the interview process will not be written in this article one by one. I'll sort out a detailed interview process and some questions you want to know ### Meituan interview experience 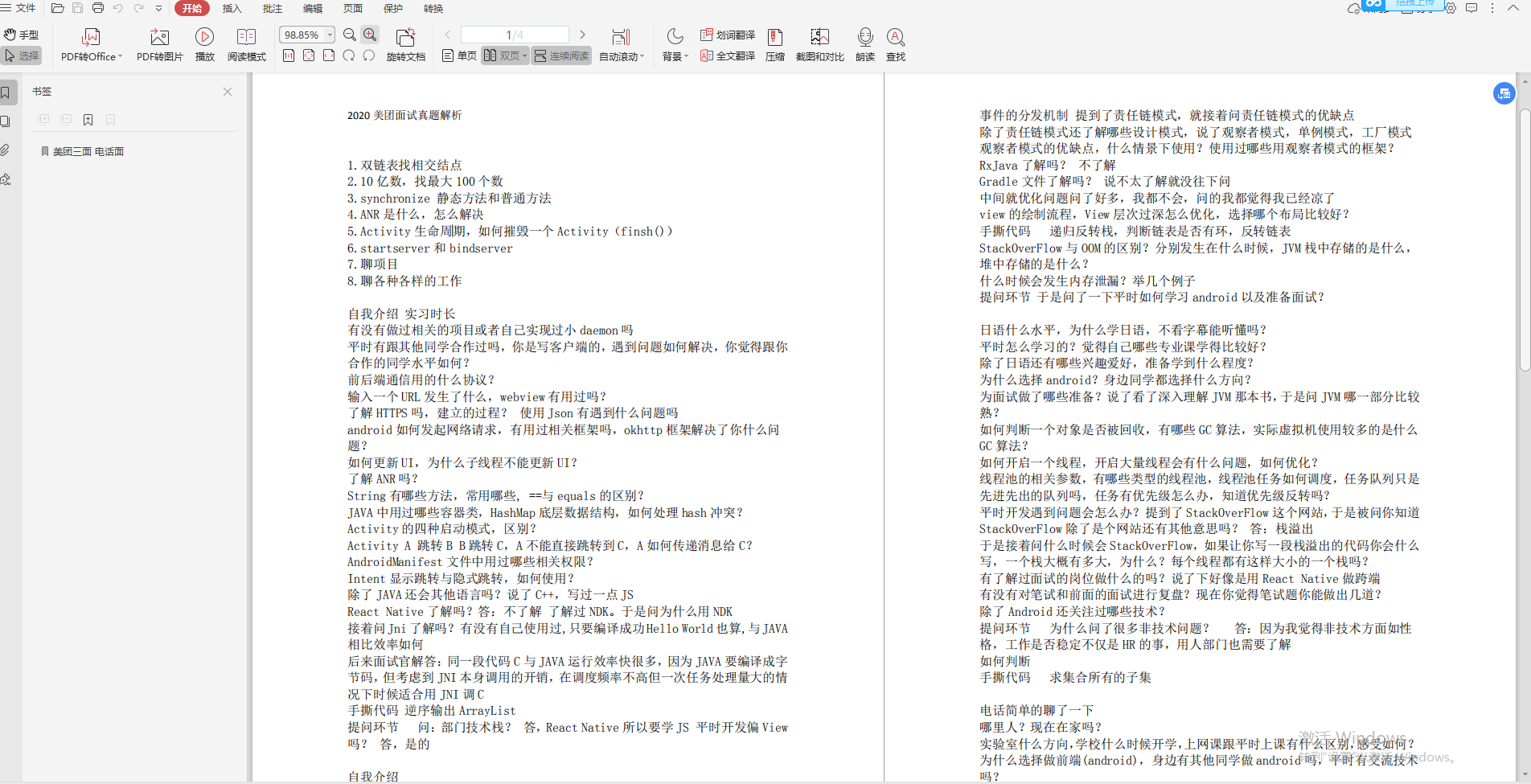 Byte interview experience 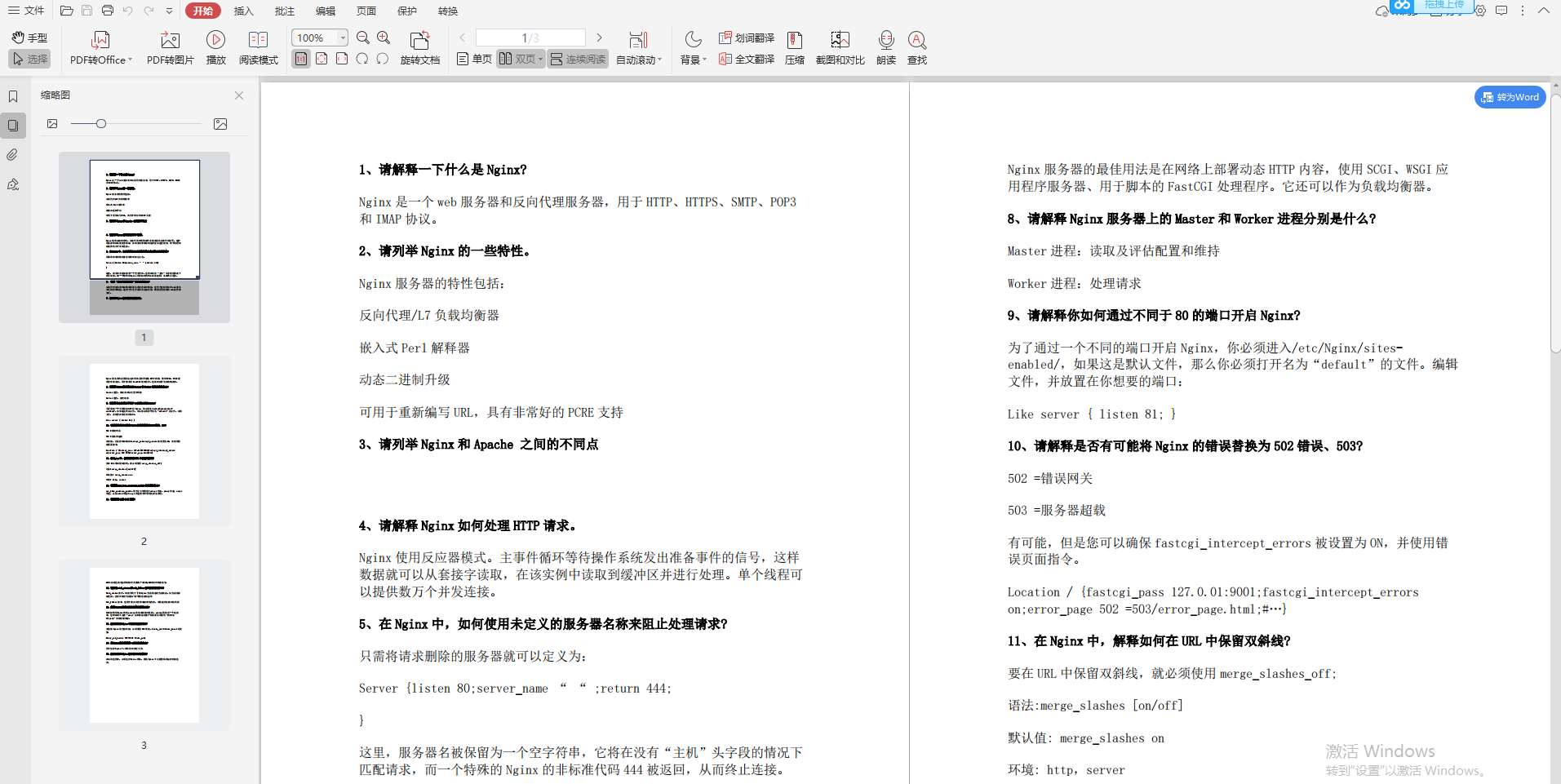 Rookie interview experience 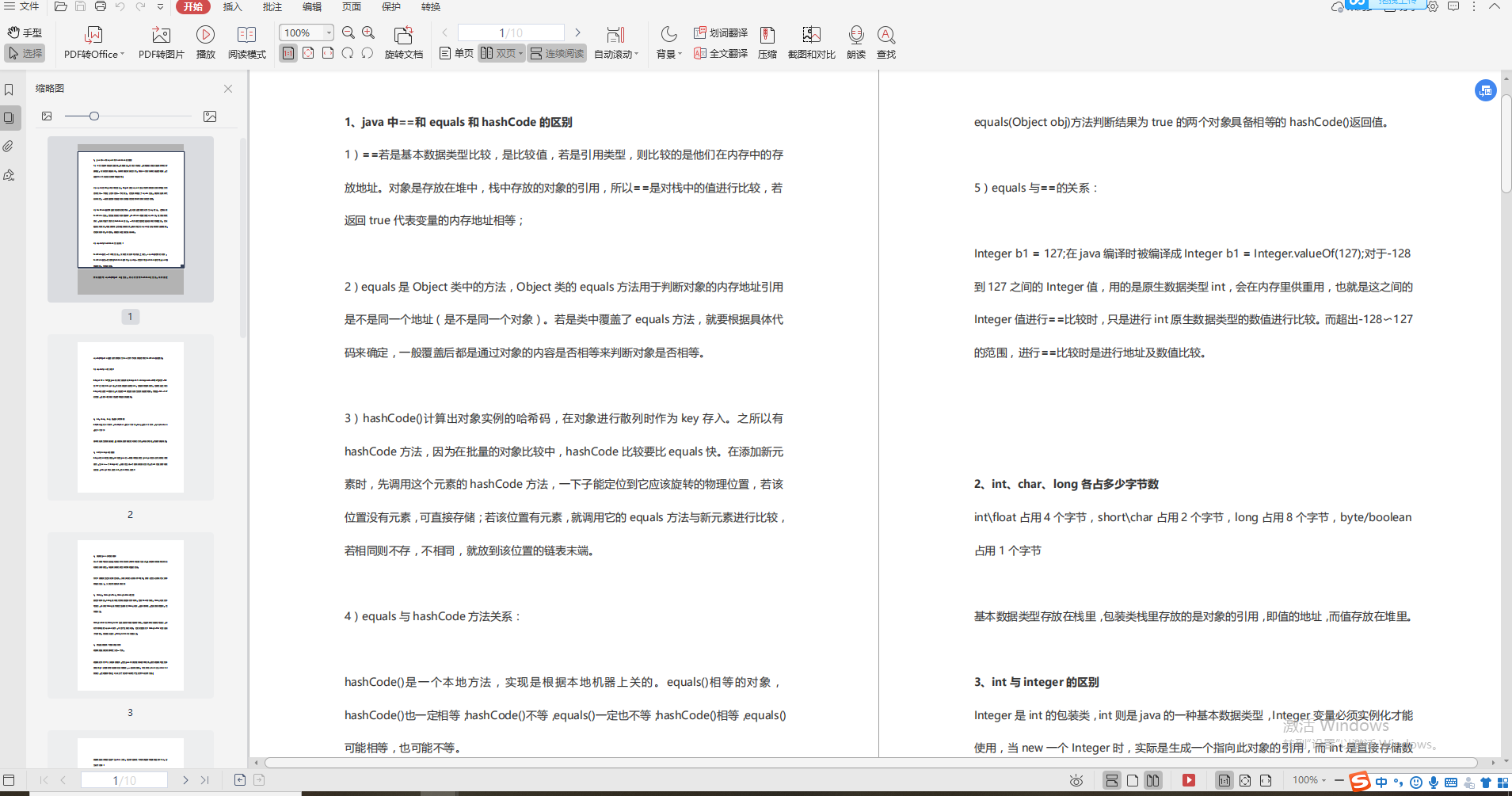 Ant gold interview experience 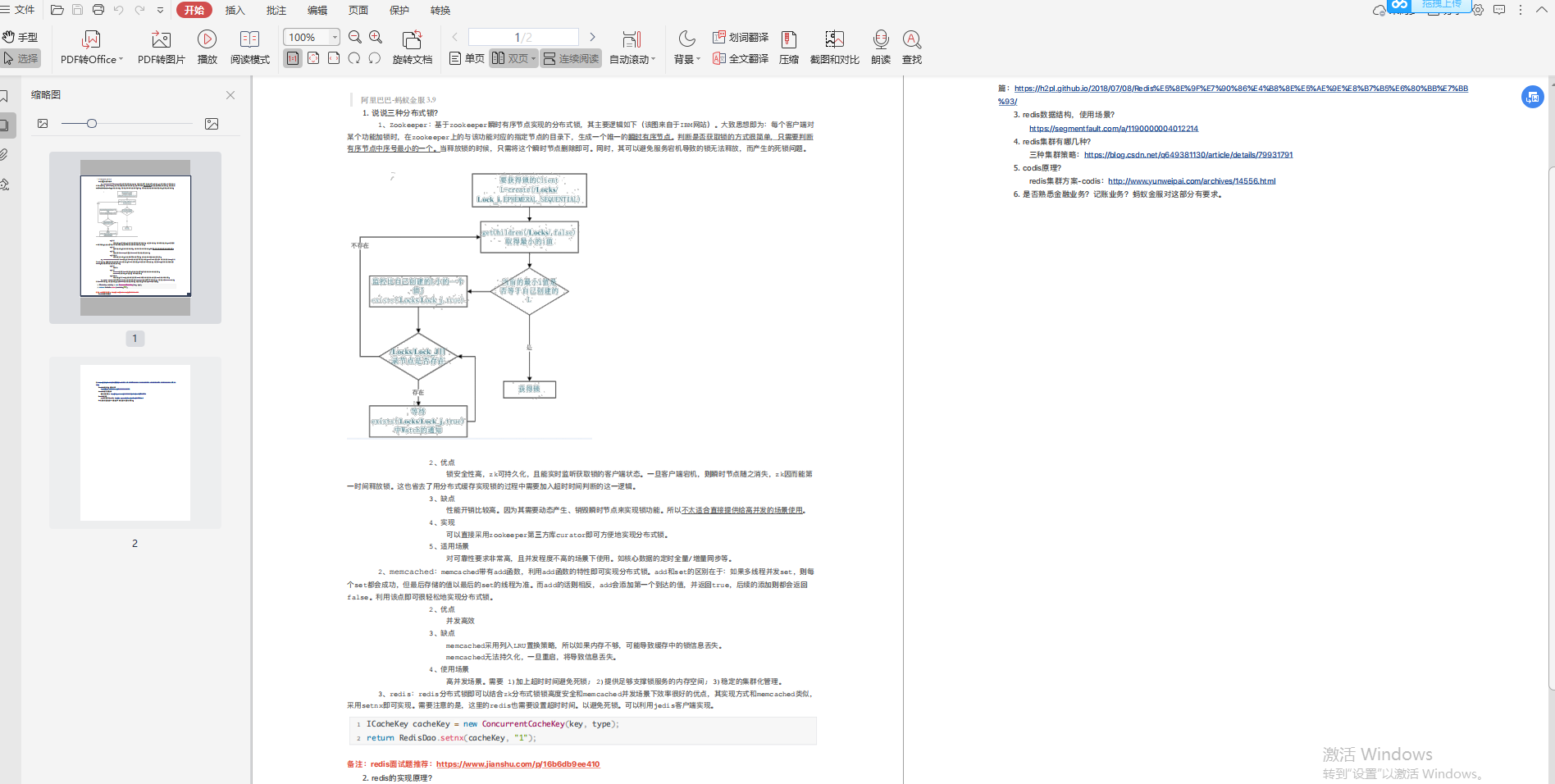 Vipshop interview experience 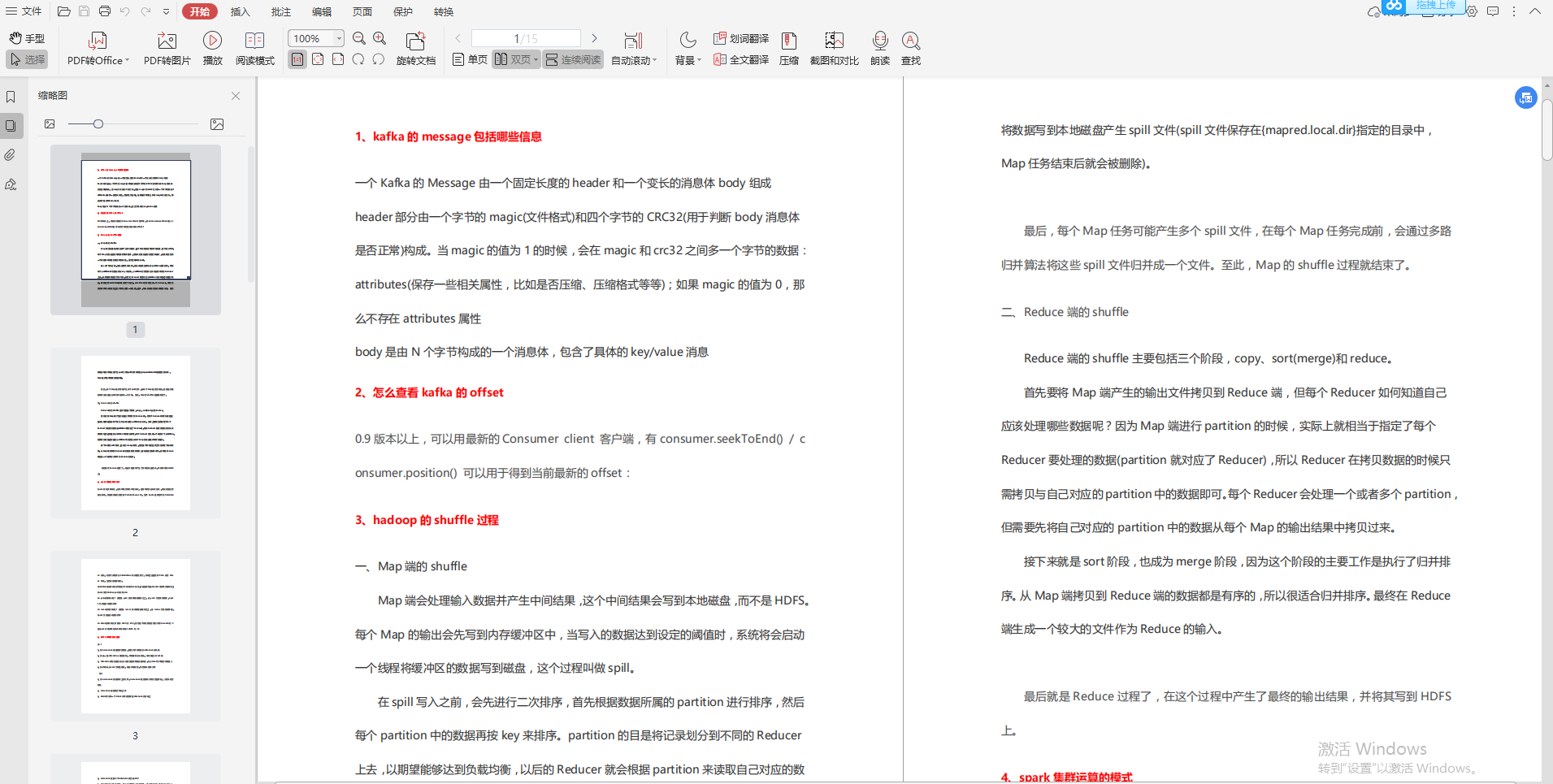 >Due to limited space, pictures and texts cannot be sent out in detail > >**[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]]( )** 631407720281)] Ant gold interview experience [External chain picture transfer...(img-mKzl7NVe-1631407720282)] Vipshop interview experience [External chain picture transfer...(img-x4YTi4zo-1631407720282)] >Due to limited space, pictures and texts cannot be sent out in detail > >**[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]]( )**