Exceptions.throwIfFatal(ex); parent.onError(ex); }
}
First create`CreateEmitter`Type of transmitter that turns downstream observer Pass it to the transmitter, note that the transmitter here is implemented`Disposable`Interface, so the transmitter will then pass through the downstream observer's`onSubscribe`Method passed to downstream observers, notice that here it is`Disposable`Object. Next, it will be given to the upstream`ObservableOnSubscribe`Add subscriptions and move downstream observer To Upstream`ObservableOnSubscribe`. To describe the subscription process, we draw a timeline: 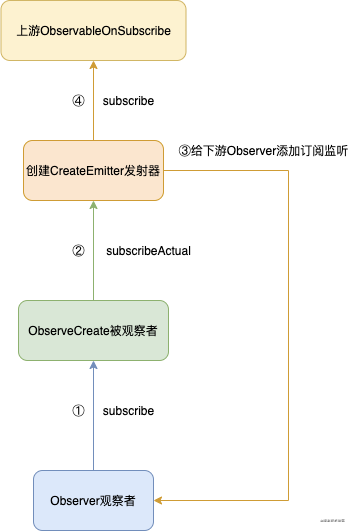 ##### []( )Summary > Subscriptions are downstream Observer Upstream Observable Send a subscription and then, during the subscription process, send it to the downstream Observer Send subscription listeners and add subscriptions to observers upstream. #### []( )send data We know above that ObservableCreate Of subscribeActual Method to upstream ObservableOnSubscribe Added onSubscribe Subscription process and transfer current transmitter to ObservableOnSubscribe,And what we defined in the example above`ObservableOnSubscribe`Internal class subscribe Method adds the following code through the transmitter passed in:
emitter.onNext(1);
emitter.onNext(2);
emitter.onNext(3);
emitter.onComplete();
So here you can see that it's through the transmitter onNext and onComplete Send data while emitter Here comes the subscription process above CreateEmitter,So look directly at it onNext and onComplete:
@Override
public void onNext(T t) {
if (t == null) { onError(ExceptionHelper.createNullPointerException("onNext called with a null value.")); return; } //If isDisposed is false, you can continue sending data if (!isDisposed()) { observer.onNext(t); }
}
It's simple, give`observer`Send data while current`observer`Is downstream from the subscription process`observer`,So you know, it's downstream in the end`observer`Received data. ##### []( )Summary > The transmitter is notified mainly by the observer upstream, and then the transmitter is sent downstream observer. ### []( )Observer Finished processing onComplete We'll be able to do it later onNext Are you?? As we can see above emitter.onNext After three times, it will send onComplete Events, that onComplete What to do:
@Override
public void onComplete() {
if (!isDisposed()) { try { observer.onComplete(); } finally { dispose(); } }
}
This is in the transmitter`onComplete`Definition,`dispose`The way is to control if you can still send data, which is `CreateEmitter`It is a`AtomicReference<Disposable>`Atomic Packaging Disposable The implementation class, and we`dispose`The method is to add a constant to the atomic class.`DISPOSED`,While in onNext Method by judgment`isDisposed`Is it false To continue sending data.`isDisposed`When is it false What? When`AtomicReference<Disposable>`Wrapper object in is not`DISPOSED`. So our`onComplete`Is used to control the inability to send data. You can pass the following code tests:
emitter.onNext(1);
emitter.onNext(2);
emitter.onComplete();
emitter.onNext(3);
Look downstream observer Can I still receive 3 more data? ##### []( )Summary > `onComplete`Is used to control the inability to send data, that is, not`onNext`Yes, including onError And can't send any more`onNext`Data, this method is also called`dispose`Method. ### []( )RxJava in map,flatMap What other operators have you used?? map and flatMap It's a conversion we use a lot. Let's look at it first map How to use:
Observable createObservable = Observable.create(new ObservableOnSubscribe() {
@Override public void subscribe(@NonNull ObservableEmitter<Integer> emitter) throws Throwable { emitter.onNext(1); emitter.onNext(2); emitter.onNext(3); emitter.onComplete(); } }); Observable<String> mapObservable = createObservable.map(new Function<Integer, String>() { @Override public String apply(Integer integer) throws Throwable { return String.valueOf(integer + 1); } }); Observer<String> observer = new Observer<String>() { @Override public void onSubscribe(@NonNull Disposable d) { Log.d(TAG, "onSubscribe:" + d.getClass().getName()); } @Override public void onNext(@NonNull String string) { Log.d(TAG, "onNext: " + string); } @Override public void onError(@NonNull Throwable e) { Log.d(TAG, "onError: " + e.getMessage()); } @Override public void onComplete() { Log.d(TAG, "onComplete"); } }; mapObservable.subscribe(observer);
}
adopt`createObservable`Of map Operation generated a`mapObservable`Observed by the`mapObservable`and`observer`Form a subscription relationship, while map Operation requires a Function Interface, the first is the input type, the second is the output type, that is apply Return value of, defined here map The type of exit is String Type. Let's see again flatMap How to use:
Observable flatMapObservable = mapObservable.flatMap(new Function<String, ObservableSource>() {
@Override public ObservableSource<Integer> apply(String s) throws Throwable { return Observable.create(new ObservableOnSubscribe<Integer>() { @Override public void subscribe(@NonNull ObservableEmitter<Integer> emitter) throws Throwable { emitter.onNext(Integer.valueOf(s)+1); emitter.onComplete(); } }); }
});
flatMapObservable.subscribe(observer);
On top mapObservable Through flatMap Return flatMapObservable,Last Passed flatMapObservable Subscribe observer. flatMap Of Function The second generic is ObservableSource Of type, Observable The parent of is ObservableSource Type, so the second parameter returns Observable It's fine too. **You can see from above map Returns another data type from the original data type, while flatMap Returns another observer through the original data type.** Questions about interviews flatMap and concatMap Here's an example to illustrate the difference:
Observable createObservable = Observable.just("1", "2", "3", "4", "5", "6", "7", "8", "9");
Observable flatMapObservable = createObservable.flatMap(new Function<String, ObservableSource>() {
@Override public ObservableSource<Integer> apply(String s) throws Throwable { if (s.equals("2")) { return Observable.create(new ObservableOnSubscribe<Integer>() { @Override public void subscribe(@NonNull ObservableEmitter<Integer> emitter) throws Throwable { emitter.onNext(Integer.valueOf(s) + 1); emitter.onComplete(); } }).delay(500, TimeUnit.MILLISECONDS); } else { return Observable.create(new ObservableOnSubscribe<Integer>() { @Override public void subscribe(@NonNull ObservableEmitter<Integer> emitter) throws Throwable { emitter.onNext(Integer.valueOf(s) + 1); emitter.onComplete(); } }); } }
});
Observable observeOnObservable = flatMapObservable.subscribeOn(Schedulers.io()).observeOn(AndroidSchedulers.mainThread());
Observer observer = new Observer() {
@Override public void onSubscribe(@NonNull Disposable d) { Log.d(TAG, "onSubscribe:" + d.getClass().getName()); } @Override public void onNext(@NonNull Integer string) { Log.d(TAG, "onNext: " + string); } @Override public void onError(@NonNull Throwable e) { Log.d(TAG, "onError: " + e.getMessage()); } @Override public void onComplete() { Log.d(TAG, "onComplete"); }
};
observeOnObservable.subscribe(observer);
On top`flatMap`For demonstration during operation`flatMap`and`concatMap`The difference between returned values when the data is 2`observable`500 milliseconds delay, we see the following results: 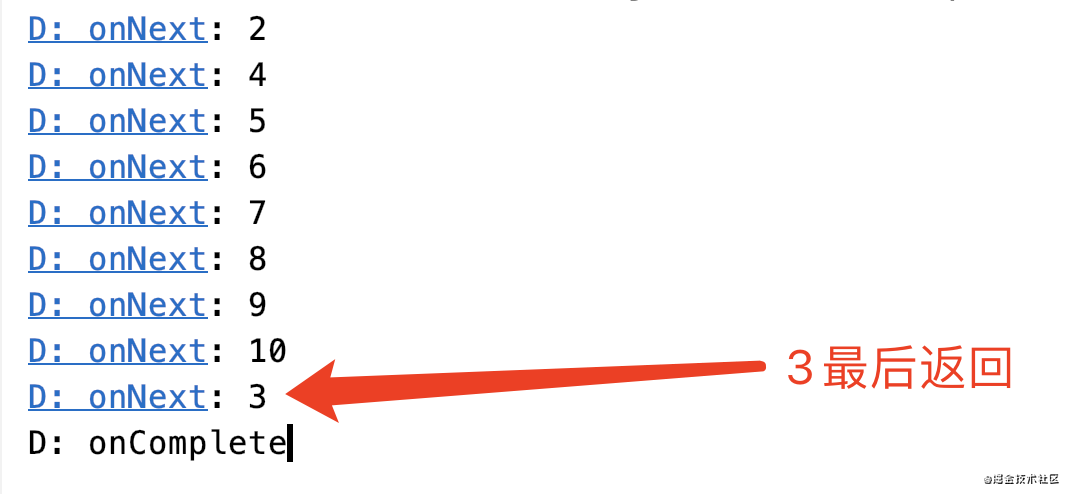 In the example above, 3 was sent from 2, which delayed 500 milliseconds when it happened to be 2. concatMap The result is returned in the order in which the data was sent. **concatMap and flatMap Function is the same, will send a data of Observable Transform to Multiple Observables,Then put the data they send into a separate Observable. It's just the last merge ObservablesflatMap Adopted merge,and concatMap Connections are used(concat). One sentence in total,The difference between them is: concatMap Is ordered, flatMap Is out of order, concatMap The order of the final output is the same as that of the original sequence, while flatMap Not necessarily, there may be interlacing.** About other operators such as merge,concat,zip They are mergers. interval Is periodic execution, timer Delayed sending of data. If you want to learn more operators, please[Stamp Official Website]( ) ### []( )Maybe,Observer,Single,Flowable,Completable The difference between several observers and in what scenarios do they work? Actually, to know the difference, let's look directly at the corresponding Observer What are the methods: * Maybe Maybe Literally means**Probably**Meaning, look at`MaybeObserver`Interface:
public interface MaybeObserver<@NonNull T> {
void onSubscribe(@NonNull Disposable d); void onSuccess(@NonNull T t); void onError(@NonNull Throwable e); void onComplete();
}
**It doesn't onNext Method, that is, you cannot send more than one data if you call back to`onSuccess`No more messages, if callback directly`onComplete`Equivalent to no data, that is Maybe Data may not be sent, if it is sent, only a single piece of data will be sent.** * Observer **Needless to say, it can send multiple pieces of data until it is sent`onError`or`onComplete`No more data will be sent. Of course, it can also be sent directly without sending data.`onError`or`onComplete`. ** * Single
public interface SingleObserver<@NonNull T> {
Last
For Android programmers, I've compiled some stuff here, including not limited to advanced UI, performance optimization, architect courses, NDK, hybrid development (ReactNative+Weex)All aspects of Android advanced practice technology such as WeChat applet, Flutter, etc. I hope to help you, also save you time to search for information on the Internet to learn, or share the dynamics to friends around you to learn together!
**[CodeChina Open Source Project: Android Learning Notes Summary + Mobile Architecture Video + Factory Interview True Topics + Project Actual Source]()**
Previous Android Advanced Architecture materials, source code, notes, videos. Advanced UI, performance optimization, architect courses, hybrid development (ReactNative+Weex) all aspects of advanced Android practice technology, there are also technology bulls in the group to discuss and communicate solutions.
This article has been Tencent CODING Open Source Hosted Project: "Android Learning Notes Summary + Mobile Architecture Video + Factory Interview True Topic + Project Actual Source" Included, self-study resources and continuous updates of series of articles...
Advanced Android practices in performance optimization, architect courses, NDK, ReactNative+Weex Wechat applets, Flutter and more; I hope to help you, save you time searching for information on the web, and share the dynamics with friends around you!**
)**
Previous Android Advanced Architecture materials, source code, notes, videos. Advanced UI, performance optimization, architect courses, hybrid development (ReactNative+Weex) all aspects of advanced Android practice technology, there are also technology bulls in the group to discuss and communicate solutions.
[img-SU0VlE8L-1631203780264)]
[img-jc72jdJa-1631203780266)]
This article has been Tencent CODING Open Source Hosted Project: "Android Learning Notes Summary + Mobile Architecture Video + Factory Interview True Topic + Project Actual Source" Included, self-study resources and continuous updates of series of articles...